To create new app "blog", type
C:\Python24\Django-0.91\django\bin\myblog>python manage.py startapp blog

Now, edit the models file(means myblog\blog\models\blog.py) for this blog, so it look like this,
from django.core import meta
class Tag(meta.Model):
slug = meta.SlugField(
'Slug',
prepopulate_from=("title",),
help_text='Automatically built from the title.',
primary_key='True'
)
title = meta.CharField('Title', maxlength=30)
description = meta.TextField(
'Description',
help_text='Short summary of this tag'
)
def __repr__(self):
return self.title
def get_absolute_url(self):
return "/tag/%s/" % self.slug
class META:
admin = meta.Admin(
list_display = ('slug', 'title',),
search_fields = ('title', 'description',),
)
class Post(meta.Model):
slug = meta.SlugField(
'Slug',
prepopulate_from=('title',),
help_text='Automatically built from the title.',
primary_key='True'
)
assoc_tags = meta.ManyToManyField(Tag)
title = meta.CharField('Title', maxlength=30)
date = meta.DateTimeField('Date')
image = meta.ImageField(
'Attach Image',
upload_to='postimgs',
blank=True
)
body = meta.TextField('Body Text')
def __repr__(self):
return self.title
def get_absolute_url(self):
return "/blog/%s/%s/" % (self.date.strftime("%Y/%b/%d").lower(), self.slug)
class META:
admin = meta.Admin(
list_display = ('slug', 'title', 'date'),
search_fields = ('title', 'description'),
date_hierarchy = ('date',),
)
ordering = ('-date',)
In the urls.py file uncomment the admin line, so the whole script look like this:
from django.conf.urls.defaults import *
urlpatterns = patterns('',
(r'^admin/', include('django.contrib.admin.urls.admin')),
)
If you get an error complaining that some files are not found in the install app after you runserver and try to connect to the admin pageels file, that is because we still haven't modify the settings.py under myblog, so that the installed application did show myblog.blog, as below.
INSTALLED_APPS = ('myblog.blog',)
Try connecting to the admin page again, get this error,
Starting server on port 8000 with settings module 'myblog.settings'.
Go to http://127.0.0.1:8000/ for Django.
Quit the server with CONTROL-C (Unix) or CTRL-BREAK (Windows).
Validating models...
Unhandled exception in thread started by
Traceback (most recent call last):
File "c:\python24\lib\site-packages\django-0.91-py2.4.egg\django\core\manageme
nt.py", line 757, in inner_run
validate()
File "c:\python24\lib\site-packages\django-0.91-py2.4.egg\django\core\manageme
nt.py", line 741, in validate
num_errors = get_validation_errors(outfile)
File "c:\python24\lib\site-packages\django-0.91-py2.4.egg\django\core\manageme
nt.py", line 634, in get_validation_errors
import django.models
File "c:\python24\lib\site-packages\django-0.91-py2.4.egg\django\models\__init
__.py", line 13, in ?
modules = meta.get_installed_model_modules(__all__)
File "c:\python24\lib\site-packages\django-0.91-py2.4.egg\django\core\meta\__i
nit__.py", line 111, in get_installed_model_modules
mod = __import__('django.models.%s' % submodule, '', '', [''])
File "C:\Python24\Django-0.91\django\bin\myblog\..\myblog\blog\models\blog.py"
, line 3
class Tag(meta.Model):
^
SyntaxError: invalid syntax
I suspect there is nothing wrong with the code syntax, but just an extra spaces or tab cuase this problem, so try to check on the code and rearrange all the indentation again. After a few changes here and there, found out that the line class Tag(meta.Model): as well as class Post(meta.Model): should be at the same indentation level as the first line from Django.core import meta . Not sure if this is the case for everyone, but once I get this change, I am able to runserver under myblog.
Still, there is an error appear even though the server started,
Starting server on port 8000 with settings module 'myblog.settings'.
Go to http://127.0.0.1:8000/ for Django.
Quit the server with CONTROL-C (Unix) or CTRL-BREAK (Windows).
Validating models...
blog.posts: "image" field: To use ImageFields, you need to install the Python Im
aging Library. Get it at http://www.pythonware.com/products/pil/ .
1 error found.
So downloaded the Python Imaging Library 1.1.5 for Python2.4 (Windows) from PythonWare. Installation is really easy, as it is an exe file.
After installing the image library, runserver again, and connect to the login screen, couldn't login with the admin account and password created under myproject. After a few try, still stuck at this frustrating screen.

But all of a sudden it struck me that I have not create a superuser account for myblog, that is why! So,
python manage.py createsuperuser
And it will prompt for username, e-mail and password. Login again, voila...

Try on adding a few tags or posts, but somehow it fails, and return some error message saying that "blog_tags" table does not exist. So, check against the the polls tutorial, I suspect this is due to a few steps that I have missed out.
So in myblog folder, type
python manage.py sql blog
and it start to create a few tables, included the missing blog_tags table. Then
python managepy install blog
This suppose to take the output from the previous sql command and execute it in the database.
And again connect to admin, try on adding tags and posts, it works now!!
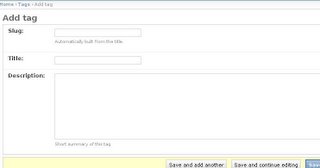
No comments:
Post a Comment