Next step will be creating a view to display some latest blog posts. Before doing so, just make sure you have added a few sample posts.
First thing to do, create a URL structure for the site. To do so, edit the urls.py file under "myblog" so it look like this.
from django.conf.urls.defaults import *
urlpatterns = patterns('',
(r'^blog/', include('myblog.blog.urls')),
((r'^admin/'), include('django.contrib.admin.urls.admin')),
)
This actually point Django to myblog\blog\urls.py, so edit myblog\blog\urls.py so it look like this,
from django.conf.urls.defaults import *
blog_dict = {
'app_label': 'blog',
'module_name': 'posts',
'date_field': 'date',
}
urlpatterns = patterns('django.views.generic.date_based',
(r'^(?P\d{4})/(?P [a-z]{3})/(?P \w{1,2})/(?P [0-9A-Za-z-]+)/$', 'object_detail', dict(blog_dict, slug_field='slug')),
(r'^(?P\d{4})/(?P [a-z]{3})/(?P \w{1,2})/$','archive_day', blog_dict),
(r'^(?P\d{4})/(?P [a-z]{3})/$', 'archive_month', blog_dict),
(r'^(?P\d{4})/$','archive_year', blog_dict),
(r'^/?$', 'archive_index', blog_dict), )
Now, in order to display this data, we have to create some templates, just as in the myproject tutorial. Edit myblog\settings.py to change the TEMPLATE_DIRS to this,
TEMPLATE_DIRS = (
"C:/Python24/Django-0.91/mytemplates/",
)
Under this "mytemplates" folder create a base.html file with this code,
<html>
<head>
<title>My First Django Blog</title>
</head>
<body>
<h1>My First Django Blog</h1>
{% block content %}Content will go here{% endblock %}
</body>
</html>
Since we have declared in myblog\urls.py the 'app_label:blog', django expect the template to be sittng in a blog folder, so create a "blog" folder in "mytemplates".
In the "blog" folder then create "posts_archive.html" and edit it so it look like this,
{% extends "base" %}
{% block content %}
<h2>Blog: Latest Postings</h2>
{% for object in latest %}
<h2><a id="{{ object.slug }}" xhref="{{ object.get_absolute_url }}">{{ object.title }}</a></h2>
{% if object.image %}<img align="right" xsrc="{{ object.get_image_url }}" /> {% endif %}
{{ object.body|truncatewords:80 }}
<div class="article_menu"><b>Posted on {{ object.date|date:"F j, Y" }}</b> Tags: {% for tag in object.get_tag_list %}{% if not forloop.first %}, {% endif %}{{ tag.title }}{% endfor %}</div>
{% endfor %}
{% endblock %}
This is actually the index for the site(this is what has been declared in the myblog\blog\urls.py as 'archive_index'), it is an archive view that display all the latest posts on the blog.
Now, connect to http://127.0.0.1:8000/blog/... we can see the beautiful bold "My First Django Blog" saying hello there...
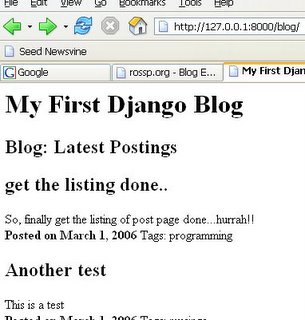
No comments:
Post a Comment